Index
Introduction
In this project, we will build an automatic night lamp using an LDR Sensor and an ESP32. When the surrounding light level drops, the LED will turn ON, and when it’s bright, the LED will turn OFF.
Required Components
- ESP32 Board
- LDR Sensor
- LED
- 220Ω Resistor
- Breadboard
- Jumper Wires
Pinout
- DHT11 Sensor
- VCC: Power supply pin (3.3V to 5V)
- GND: Ground pin
- DO: Digital data output pin
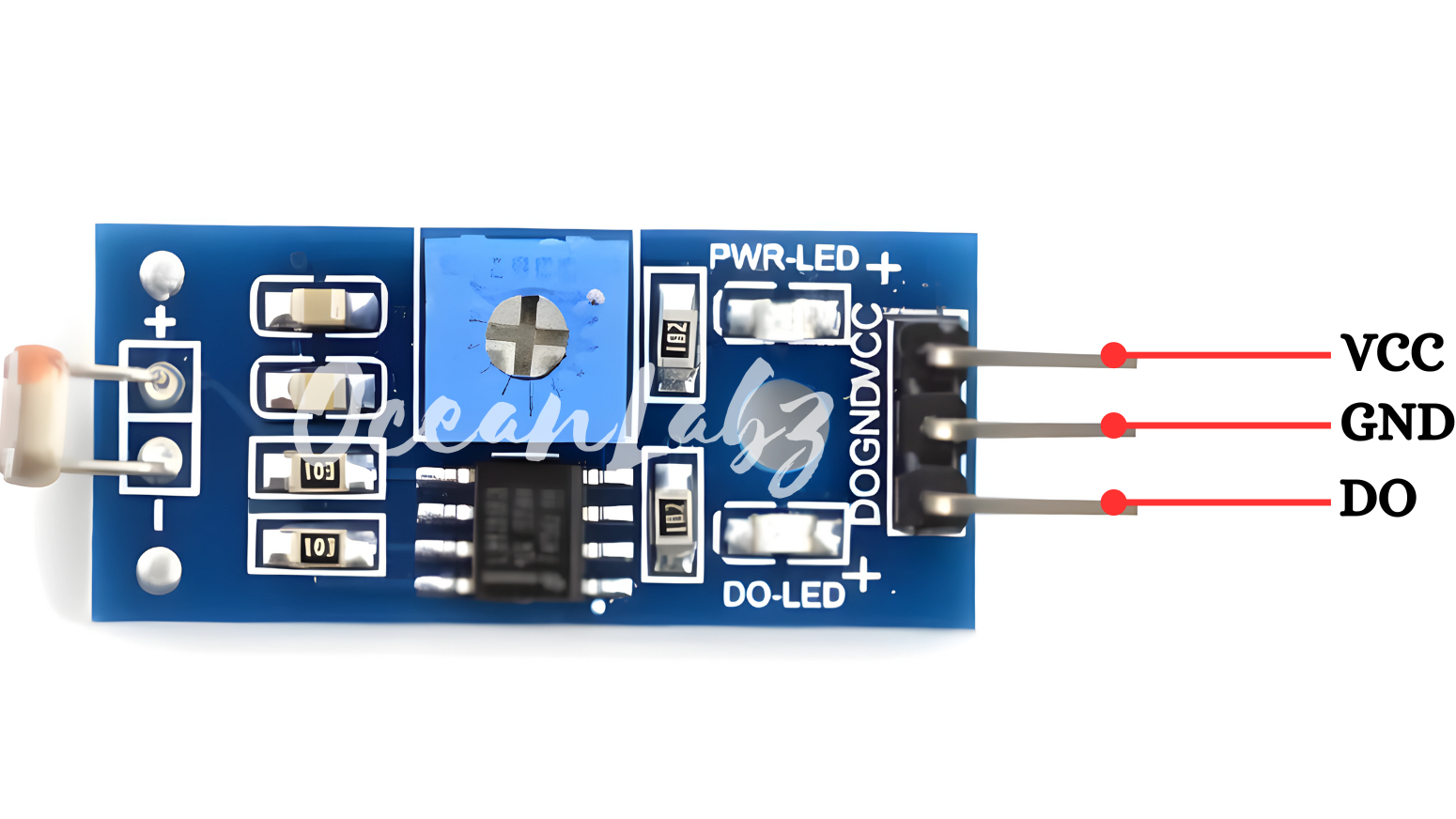
- LED
- LED Anode (+)
- LED Cathode (-)
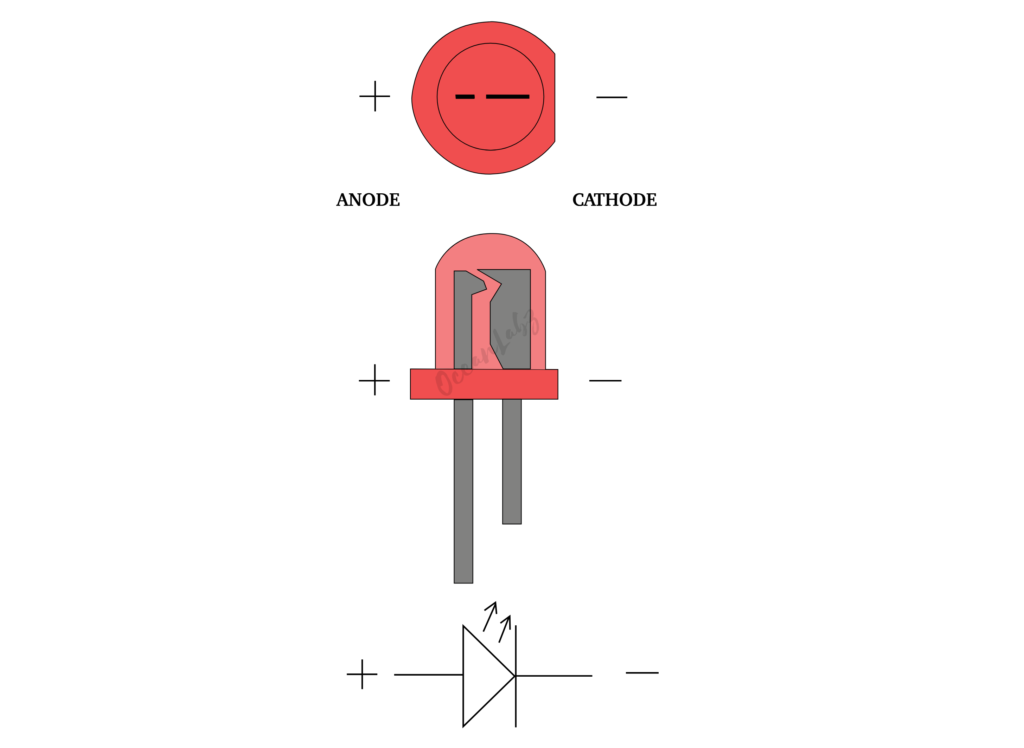
Circuit Diagram
- LDR Sensor
- LDR Sensor VCC → ESP32 3.3V
- LDR Sensor GND → ESP32 GND
- LDR Sensor DO → ESP32 GPIO 34
- LED
- Anode (Long Leg) → ESP32 GPIO 26 (via 220Ω Resistor)
- Cathode (Short Leg) → GND
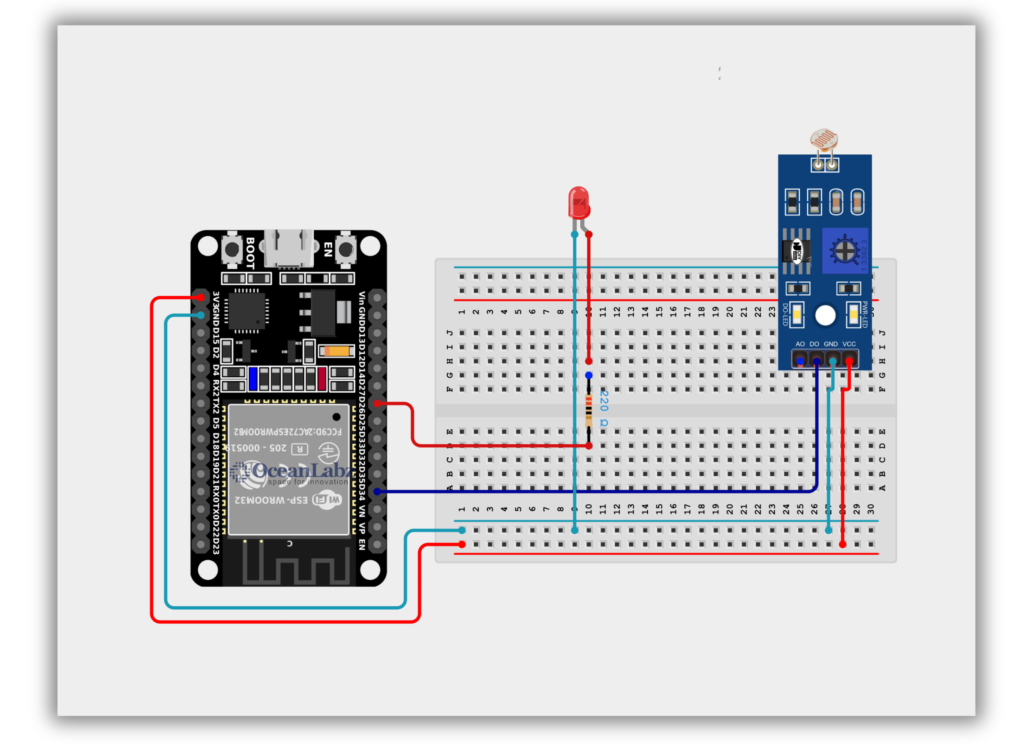
Code / Programming
/*
Filename: ol_ldr_auto_led.ino
Description: Reads LDR sensor and turns LED ON in dark and OFF in light
Author: www.oceanlabz.in
Modification: 1/4/2025
*/
#define LDR_PIN 34 // LDR connected to GPIO 34
#define LED_PIN 26 // LED connected to GPIO 26
void setup() {
pinMode(LED_PIN, OUTPUT); // Set LED pin as output
Serial.begin(115200); // Start serial communication
}
void loop() {
int ldrValue = analogRead(LDR_PIN); // Read LDR value
Serial.println(ldrValue); // Print value to Serial Monitor
if (ldrValue < 1000) {
digitalWrite(LED_PIN, HIGH); // Turn ON LED in darkness
} else {
digitalWrite(LED_PIN, LOW); // Turn OFF LED in light
}
delay(500); // Delay for half a second
}
Explanation
- LDR detects light intensity, and the ESP32 reads its analog value.
- If LDR value is low (dark environment), the LED turns ON.
- If LDR value is high (bright environment), the LED turns OFF.
Troubleshooting
- LED not turning ON? Try adjusting the threshold value (
1000
). - LDR value not changing? Check LDR connections.
- ESP32 not reading LDR values? Use a different ADC pin (like GPIO 35).
Project 1: ESP32 Laser Security Beam
Introduction
The KY-008 laser module emits a focused red laser beam, while the LDR (Light Dependent Resistor) detects changes in light intensity.
When the laser directly hits the LDR, its resistance remains low — but if the beam is interrupted, resistance increases.
With ESP32, this setup can be used for security triggers, object detection, or counting systems.
Required Components
- ESP32 Board
- Laser Module
- LDR Sensor Module
- Jumper wires
- Breadboard
Pinout
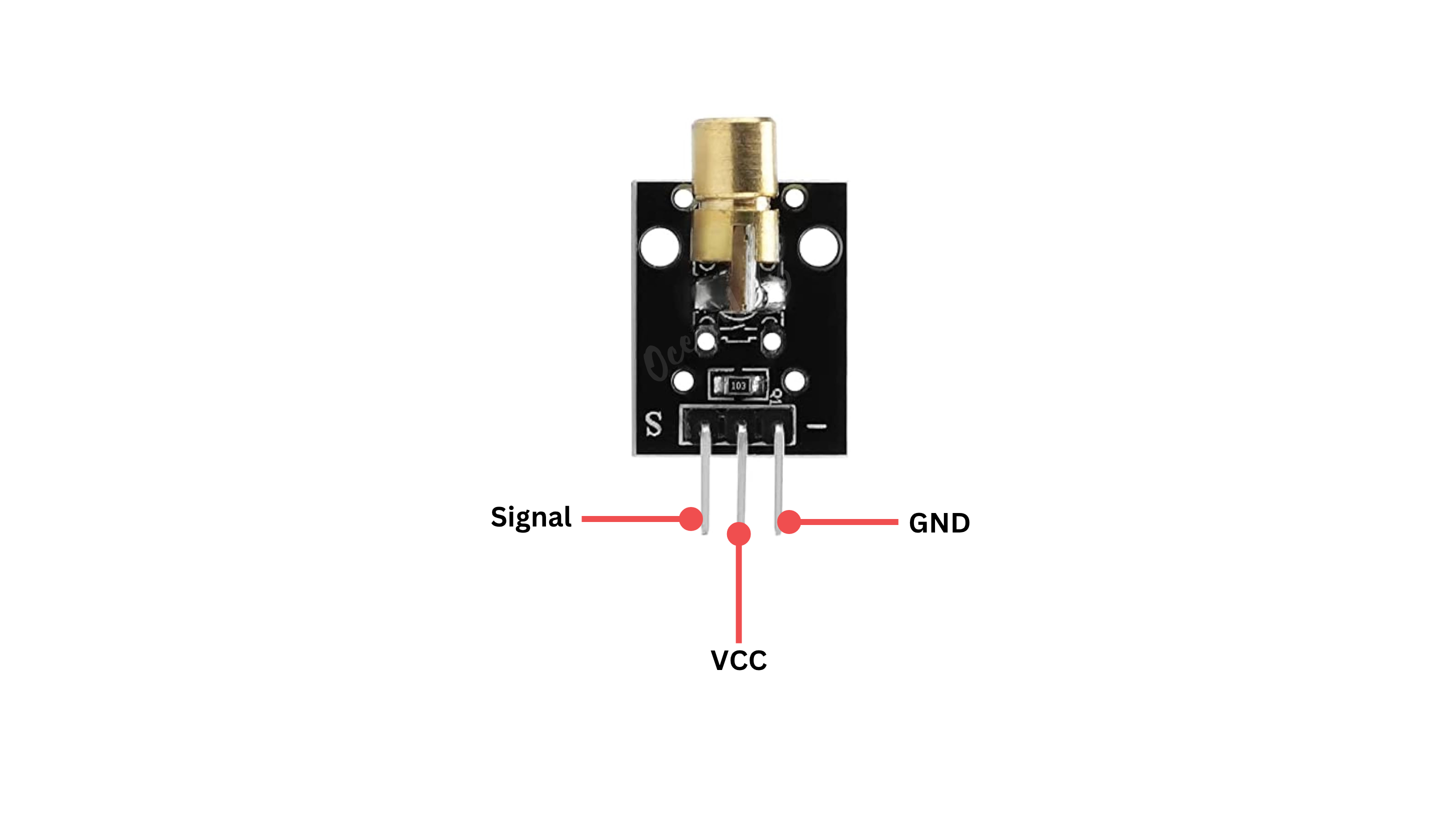
Circuit Diagram / Wiring
- Laser Module with ESP32
- Laser VCC → 3.3, 5V (ESP32)
- Laser GND → GND (ESP32)
- Laser Signal → 23 (ESP32)
- LDR Module with ESP32
- LDR VCC → 5V (ESP32)
- LDR GND → GND (ESP32)
- LDR A0 → 34 (ESP32)
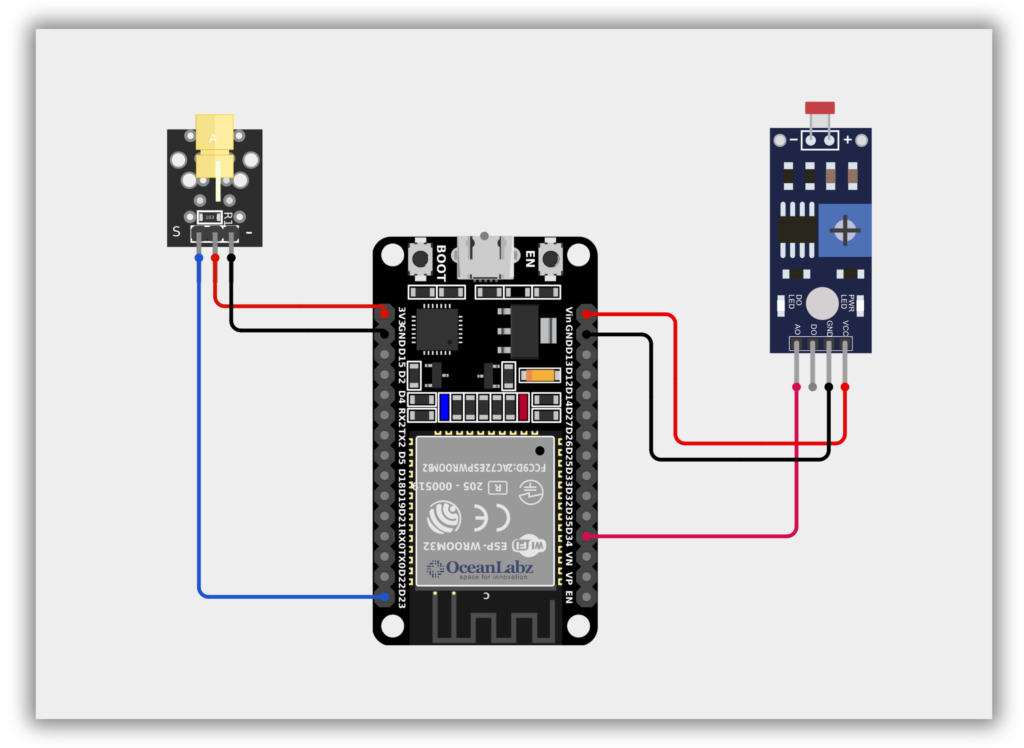
Code / Programming
/*
Filename: ol_laser_tripwire.ino
Description: Detects interruption in laser beam using KY-008 and LDR sensor
Author: www.oceanlabz.in
Modification: 1/4/2025
*/
int laserPin = 23; // Laser KY-008 connected to GPIO 23
int ldrPin = 34; // LDR connected to ADC-capable GPIO 34
void setup() {
pinMode(laserPin, OUTPUT); // Set laser pin as output
Serial.begin(115200); // Start serial communication
}
void loop() {
digitalWrite(laserPin, HIGH); // Turn on the laser
int ldrValue = analogRead(ldrPin); // Read the LDR value
Serial.print("LDR Value: ");
Serial.println(ldrValue);
// Check if laser beam is interrupted (LDR reads lower value)
if (ldrValue < 500) {
Serial.println("Laser beam interrupted!");
}
delay(200); // Wait for 200ms before the next reading
}
Explanation
- The KY-008 laser module emits a beam, which directly hits the LDR sensor under normal conditions.
- The ESP32 reads the LDR’s analog value via an ADC pin to detect light intensity.
- If the beam is interrupted (e.g., by a hand or object), the LDR value drops, triggering an alert.
Troubleshooting
- Make sure the LDR is connected to an ADC-compatible pin like GPIO34–39 (not digital-only pins).
- If values don’t change, check laser alignment, LDR sensitivity, and adjust the threshold (e.g.,
ldrValue < 500
). - If laser doesn’t light up, ensure it’s connected to a working digital pin and receiving proper voltage (3.3V or 5V).