Index
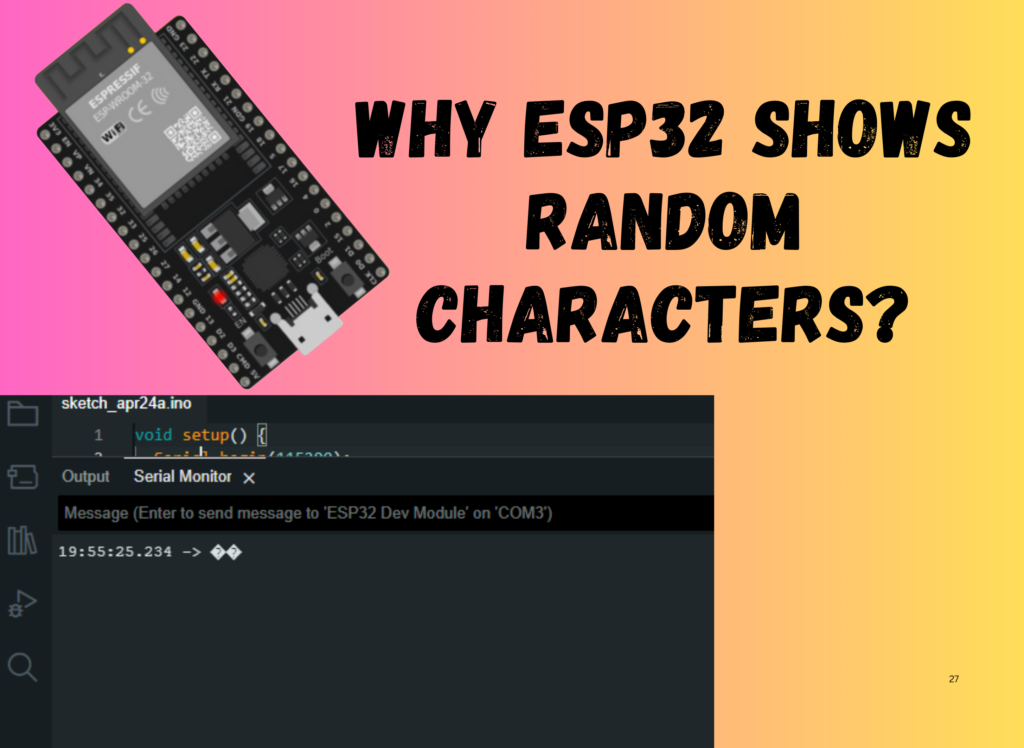
Introduction
Ever uploaded your code to the ESP32, opened the Serial Monitor, and saw a flood of random symbols like ⸮⸮⸮⸮⸮⸮
or gibberish text? Don’t worry — your ESP32 is not broken! This issue is super common and super easy to fix — once you know what’s going on.
Let’s decode the mystery behind the “garbage values” in your Serial Monitor.
What Are Garbage Values?
When you open the Serial Monitor and instead of readable text you see symbols, emojis, or random characters, that usually means one thing:
❗ The baud rate of your Serial Monitor doesn’t match the baud rate your ESP32 is using to send data.
Why Baud Rate Mismatch Causes This
Baud rate is the speed at which data is sent via serial communication (like USB). If ESP32 is sending data at 115200 bps and your Serial Monitor is listening at 9600 bps — you’ll see garbage.
It’s like someone speaking Spanish fast while you’re trying to read in English slowly — jumbled mess!
Common Baud Rates for ESP32
Here are some common baud rates used in ESP32 projects:
Use Case | Typical Baud Rate |
---|---|
Most examples & Serial.begin() default | 115200 |
Some boot messages | 74880 (rare!) |
Very simple sketches | 9600 |
ESP-NOW / OTA logs | Sometimes 250000 |
Always make sure that the value you write in your code matches the one in your Serial Monitor.
Step-by-Step Fix
Step 1: Check Your Code
Open your Arduino sketch and find this line in setup()
:
Serial.begin(115200); // Or whatever rate you are using
Make a note of the number (like 115200, 9600, etc.)
Step 2: Match the Baud Rate in Serial Monitor
In the Arduino IDE Serial Monitor, check the dropdown at the bottom right corner. Make sure it matches:
If your code says Serial.begin(115200)
→ set Serial Monitor to 115200.
Step 3: Reopen the Serial Monitor
After setting the correct baud rate, close and reopen the Serial Monitor to apply the change.
Boom! No more garbage — now you should see clean, readable output like:
Connecting to WiFi...
WiFi connected. IP address: 192.168.1.5
Still Seeing Junk? Check These
- Multiple
Serial.begin()
in code? Use only one. - Wrong board selected? Ensure “ESP32 Dev Module” is selected under Tools > Board.
- USB cable issue? Try a different USB cable — some charge-only cables don’t send data.
- Using
Serial2
orSerial1
? Make sure you’re monitoring the right port.
Bonus: Debug Boot Messages
ESP32 sometimes prints system logs before your sketch starts. These are printed at a weird baud rate: 74880.
Try setting Serial Monitor to 74880 to see early boot logs (optional, mostly for advanced debugging).
TL;DR Fix Summary
- Check your
Serial.begin(baudRate)
in code - Match Serial Monitor baud rate to it (115200 is most common)
- Reopen Serial Monitor
- Still junk? Try a new cable or board setting
Conclusion
Gibberish in the Serial Monitor can be frustrating — but the fix is usually just a click away. Always double-check your baud rate, and you’ll be back to clean debugging in seconds.